Understanding Service Contracts in Magento 2
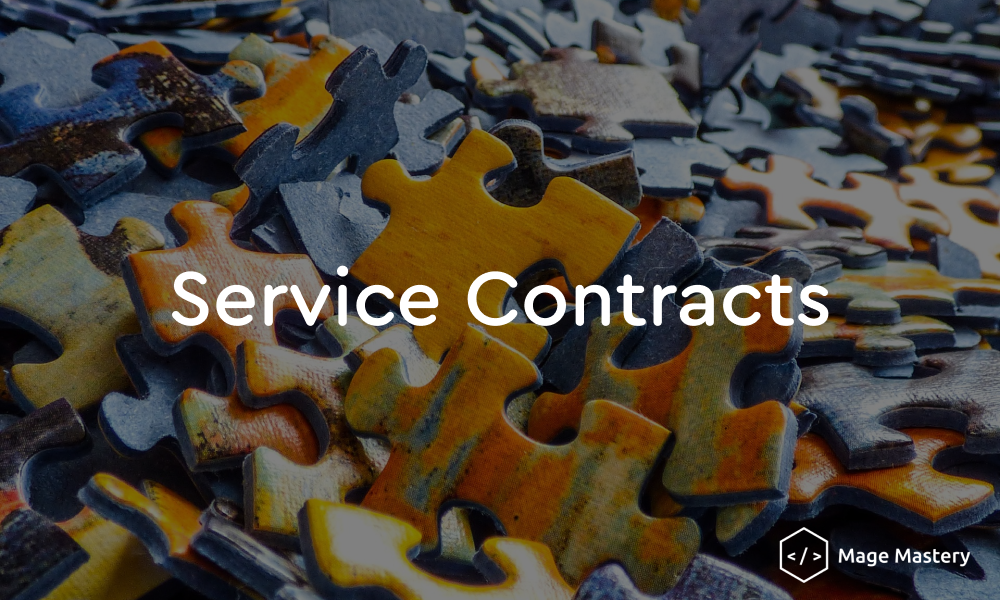
Service contracts form the backbone of robust and secure module development in Magento 2. By creating a layer between service requesters like Controllers, APIs, and third-party modules, service contracts ensure data integrity, security, and maintainability, while also enabling seamless integration and independence from major upgrades.
At their core, service contracts consist of PHP classes and interfaces that shield models, resource models, and collections from direct access by service requesters. This separation of concerns not only enhances security but also facilitates seamless integration and reduces the impact of major upgrades.
Key Components of Service Contracts
Data Interfaces
These interfaces define the methods for accessing data, ensuring data integrity and consistency across modules. In the provided example, the `BalanceInterface` defines methods for setting and getting balance-related data.
Service Interfaces
Service interfaces define the methods that service classes must implement to provide data to controllers, APIs, and third-party modules. The `StoreCreditRepositoryInterface` in the example specifies methods for fetching store credit balances.
Example
Service Interface
declare(strict_types=1); namespace MageMastery\StoreCredit\Api; use Magento\Framework\Exception\NoSuchEntityException; interface StoreCreditRepositoryInterface { /** * @param \Magento\Framework\Api\SearchCriteriaInterface $searchCriteria * @return \Pronko\MageMastery\Api\Data\BalanceSearchResultInterface */ public function geList(\Magento\Framework\Api\SearchCriteriaInterface $searchCriteria): \MageMastery\StoreCredit\Api\Data\BalanceSearchResultInterface; /** * Get Store Credit balance for the customer. * * @param int $customerId * @return \MageMastery\StoreCredit\Api\Data\BalanceInterface */ public function getBalance(int $customerId): \MageMastery\StoreCredit\Api\Data\BalanceInterface; }
Data Interface
declare(strict_types=1); namespace Pronko\StoreCredit\Api\Data; use Magento\Framework\Api\ExtensibleDataInterface; interface BalanceInterface extends ExtensibleDataInterface { /** * Set balance id * * @param int $balanceId * @return void */ public function setBalanceId(int $balanceId): void; /** * Get balance id * * @return int */ public function getBalanceId(): int; /** * Set balance * * @param float $balance * @return void */ public function setBalance(float $balance): void; /** * Get balance * * @return float */ public function getBalance(): float; /** * Set customer ID * * @param int $customerId * @return void */ public function setCustomerId(int $customerId): void; /** * Get customer ID * * @return int */ public function getCustomerId(): int; }
In the provided example, the service interface StoreCreditRepositoryInterface
defines methods for retrieving store credit balances using search criteria and customer ID. The associated data interface BalanceInterface
defines methods for setting and getting balance-related data.
Benefits of Service Contracts
- Independence from Magento Upgrades: By decoupling service requesters from underlying data models, service contracts ensure that modules remain unaffected by major upgrades to Magento. This increases module durability and reduces maintenance overhead.
- Enhanced Security: Service contracts restrict direct access to sensitive data, thereby enhancing security and mitigating the risk of data breaches.
- Consistency and Reliability: By defining clear interfaces and separation of concerns, service contracts promote code consistency and reliability, leading to better maintainability and scalability of Magento applications.
Conclusion
In conclusion, service contracts serve as a powerful tool in Magento 2 development, providing security, reliability, and consistency to modules and their APIs. By adhering to service contract principles, developers can build robust, secure, and future-proof Magento applications.